Note: This guide is now deprecated.
Did you install Node (NodeJS) on your Ubuntu or Debian server, only to find that it installed an older version of Node? Do you require Node v18 but only have Node v12 or other? Are you having trouble starting MongoDB or connecting with Mongoose? Follow along for the solution.
If you try to install Mongoose modules using an older version of Node, you may encounter an error like this:
npm WARN EBADENGINE Unsupported engine {
npm WARN EBADENGINE package: 'mongoose@7.0.1',
npm WARN EBADENGINE required: { node: '>=14.0.0' },
npm WARN EBADENGINE current: { node: 'v12.22.12', npm: '7.5.2' }
npm WARN EBADENGINE }
npm WARN EBADENGINE Unsupported engine {
npm WARN EBADENGINE package: 'bson@5.0.1',
npm WARN EBADENGINE required: { node: '>=14.20.1' },
npm WARN EBADENGINE current: { node: 'v12.22.12', npm: '7.5.2' }
npm WARN EBADENGINE }
npm WARN EBADENGINE Unsupported engine {
npm WARN EBADENGINE package: 'mongodb@5.1.0',
npm WARN EBADENGINE required: { node: '>=14.20.1' },
npm WARN EBADENGINE current: { node: 'v12.22.12', npm: '7.5.2' }
npm WARN EBADENGINE }
npm WARN EBADENGINE Unsupported engine {
npm WARN EBADENGINE package: 'mquery@5.0.0',
npm WARN EBADENGINE required: { node: '>=14.0.0' },
npm WARN EBADENGINE current: { node: 'v12.22.12', npm: '7.5.2' }
npm WARN EBADENGINE }
That’s because by default the Ubuntu repositories don’t include the official Node one.
Update the system
Connect to your server via SSH or open a terminal if you’re logged in locally. Update your package list and system:
sudo apt update && apt upgrade -y
Install prerequisites
Install the required dependencies:
sudo apt install wget gnupg -y
Add MongoDB repo
wget -qO - https://www.mongodb.org/static/pgp/server-6.0.asc | sudo apt-key add -echo "deb [ arch=amd64,arm64 ] https://repo.mongodb.org/apt/debian buster/mongodb-org/6.0 main" | sudo tee /etc/apt/sources.list.d/mongodb-org-6.0.listsudo apt update
Install & enable MongoDB
sudo apt install -y mongodb-orgsudo systemctl start mongodsudo systemctl enable mongod
Install Node 18 and NPM 9
curl -fsSL https://deb.nodesource.com/setup_18.x | sudo bash -sudo apt-get install -y nodejs
Test out Mongoose
mkdir mongoose-test && cd mongoose-test
Add the follow code to test.js using your favorite editor
nano test.js
const express = require('express');
const mongoose = require('mongoose');
const app = express();
// Connect to MongoDB
mongoose.connect('mongodb://localhost/my_database', { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('Connected to MongoDB'))
.catch(err => console.error('Error connecting to MongoDB:', err));
// Define a simple schema
const userSchema = new mongoose.Schema({
name: String,
age: Number
});
// Create a model based on the schema
const User = mongoose.model('User', userSchema);
// Define route
app.get('/', (req, res) => {
res.send('Welcome to the Mongoose app!');
});
app.listen(3000, () => {
console.log('App listening on port 3000');
});
Save the file, and initialize NPM and install requires modules:
npm init -ynpm i express mongoose
Run the app:
node test.js

If you see “Connected to MongoDB” then you have successfully installed MongoDB on Debian 11
Interacting with MongoDB
Starting from MongoDB 5.0, the MongoDB can be interacted with using the mongosh
shell.
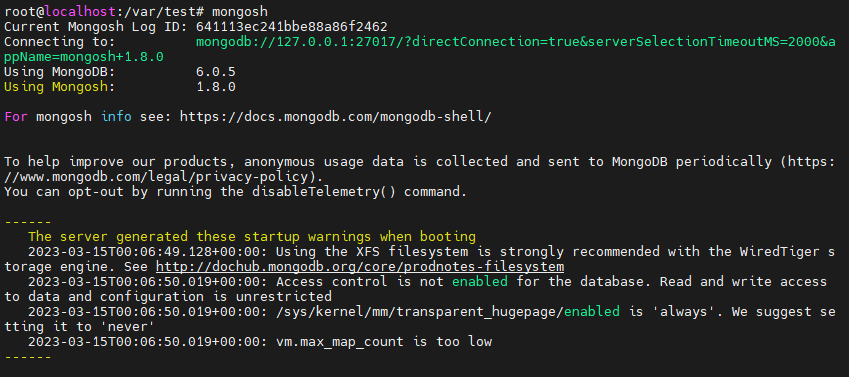
You can perform CRUD operations, run administrative commands, and execute JavaScript code in the shell. Once connected to the mongosh
shell, you can perform various operations:
Show available databases:
show dbs
Switch to a specific database or create a new one:
use myDatabase
Show available collections in the current database:
show collections
Insert a document into a collection:
db.myCollection.insertOne({ name: "John Doe", age: 30 })
Query documents from a collection (in this case, print all documents):
db.myCollection.find()
Update a document in a collection:
db.myCollection.updateOne({ name: "John Doe" }, { $set: { age: 31 } })
Delete a document from a collection:
db.myCollection.deleteOne({ name: "John Doe" })
Run administrative commands, like creating an index:
db.myCollection.createIndex({ name: 1 })
To exit the mongo shell, type exit
or press Ctrl + C
.
Using screen to run multiple apps via SSH
You can use the screen
command to run multiple terminal sessions within a single shell session, which can be useful for bouncing between your mongosh shell and Node.js app.
Install screen
sudo apt install screen
To start a new screen session, run the command:
screen
Once you are in a screen session, you can start your MongoDB shell by running the mongosh
command.
To detach from the current screen session and return to the original shell session, press the CTRL+a
keys, followed by the d
key.
To resume a detached screen session, run the command:
screen -r
To create a new screen window within the current session, press the CTRL+a
keys, followed by the c
key.
You can switch between screen
windows by pressing the CTRL+a
keys, followed by the n
or p
key to move to the next or previous window, respectively.
To exit a screen
session, you can use the exit
command.
Using screen
can help you easily switch between your MongoDB terminal and Node.js app without needing to open multiple terminal windows or tabs.